Why Extension Method: Another question is, why do we need to use the extension methods, we can create our own methods in client application or in other class library and we can call those methods from the client application but the important point is, these methods would not be the part of that class (class of the third party dll). So adding the extension methods in the class, you are indirectly including another behavior in that class. I am talking about the class which is the part of the third party dll (Most Important).
So when you create your own extension methods for specific types those methods would be accessible for that type.
This can be easily understood by the following diagram:
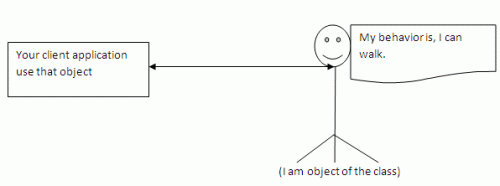
(I am object of the class)
After some time, client application wants to add another behavior in that class; let's say 'talk' then extension methods can be used to add this extra behavior in that class without altering any code.
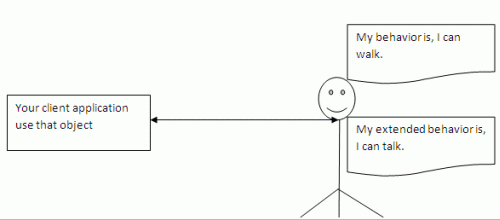
Implementation: The following is the structure of the third party class, which is not accessible to the developer. This class contains a method 'Walk'.public class Class1{
public String Walk()
{ return "I can Walk"; }}
So when you create your own extension methods for specific types those methods would be accessible for that type.
This can be easily understood by the following diagram:
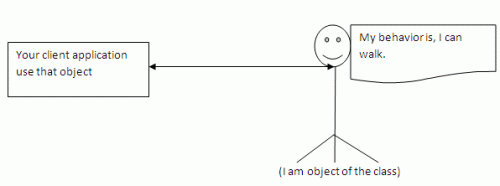
(I am object of the class)
After some time, client application wants to add another behavior in that class; let's say 'talk' then extension methods can be used to add this extra behavior in that class without altering any code.
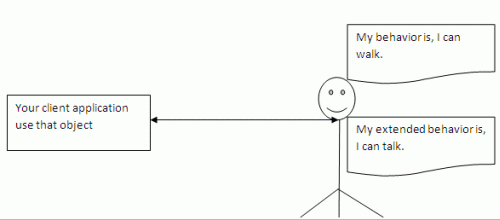
Implementation: The following is the structure of the third party class, which is not accessible to the developer. This class contains a method 'Walk'.public class Class1{
public String Walk()
{ return "I can Walk"; }}
Now it's time to create the Extension Method. Just use the following steps:
Step 1: Create the Static Class
Step 2: Create the Static Method and give any logical name to it.
Step 3: In the Extension Method Parameter, just pass this along with the object type and
the object name as given below:
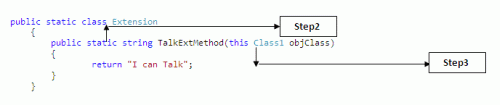
The client application can access both the method "Walk" and the extension method "TalkExtMethod":Class1 obj = new Class1 ();
Step 1: Create the Static Class
Step 2: Create the Static Method and give any logical name to it.
Step 3: In the Extension Method Parameter, just pass this along with the object type and
the object name as given below:
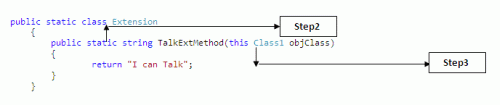
The client application can access both the method "Walk" and the extension method "TalkExtMethod":Class1 obj = new Class1 ();
Obj.TalkExtMethod ()
obj.Walk ()
Benefits: There may be various benefits of using the Extension Methods in your code. Let's say you are using the method1 () of the class in the third party dll and the object instantiation has failed due to another reason; then you will get the object reference error while calling the method1 () if you did not handle the null reference check.Class1 obj = GetClass1Object ();//If the obj is null, you will get the object reference errorobj.Method1();
Although the following code is safer than previous one but the problem is you will have to include this check every time when you call the Method1 and if the developer forgets to include this check in the code then the program throws an object reference error.Class1 obj = GetClass1Object ();if (obj!= null)
{
obj.Method1 ();
}
If we use the extension method to solve this problem we get outstanding results. Instead of checking the null reference every time in the code we handle it in the extension methods which is called by the client application.public static string Method1ExtMethod(this Class1 objClass)
{ //If the obj is not null, then call the Method1()
obj.Walk ()
Benefits: There may be various benefits of using the Extension Methods in your code. Let's say you are using the method1 () of the class in the third party dll and the object instantiation has failed due to another reason; then you will get the object reference error while calling the method1 () if you did not handle the null reference check.Class1 obj = GetClass1Object ();//If the obj is null, you will get the object reference errorobj.Method1();
Although the following code is safer than previous one but the problem is you will have to include this check every time when you call the Method1 and if the developer forgets to include this check in the code then the program throws an object reference error.Class1 obj = GetClass1Object ();if (obj!= null)
{
obj.Method1 ();
}
If we use the extension method to solve this problem we get outstanding results. Instead of checking the null reference every time in the code we handle it in the extension methods which is called by the client application.public static string Method1ExtMethod(this Class1 objClass)
{ //If the obj is not null, then call the Method1()
if (objClass! = null)
{
return objClass.Method1 ();
}
return string.Empty;
}
{
return objClass.Method1 ();
}
return string.Empty;
}
Class1 obj = GetClass1Object ();//Don't include any check whether the object is null or notobj.Method1ExtMethod()
What is Extension Methods
1.Extension methods allow an existing type to be extended with new methods without altering the definition of the original type.
2.An extension method is a static method of a static class, where the this modifier is applied to the first parameter.
3.The type of the first parameter will be the type that is extended.
Example:
public static class StringHelper
{
public static bool IsCapitalized (this string s)
{
if (string.IsNullOrEmpty(s)) return false;
return char.IsUpper (s[0]);
}
}
4.The IsCapitalized extension method can be called as though it were an instance method on a string.
String s = "Perth“;
Console.WriteLine (s.IsCapitalized());
5.An extension method call, when compiled, is translated back into an ordinary static method call.
Console.WriteLine (StringHelper.IsCapitalized (s));
Ambiguity and Resolution about Extension Methods
1.An extension method cannot be accessed unless the namespace is in scope.
2.Any compatible instance method will always take precedence over an extension method.
3. If two extension methods have the same signature, the extension method must be called as an ordinary static method to disambiguate the method to call.
4.If one extension method has more specific arguments, however, the more specific method takes precedence.
Example :-
static class StringHelper
{
public static bool IsCapitalized (this string s) {...}
}
static class ObjectHelper
{
public static bool IsCapitalized (this object s) {...}
}
bool test1 = "Perth".IsCapitalized();
bool test2 = (ObjectHelper.IsCapitalized ("Perth"));